Secure Your Computer: How to Build a Python Port Checker
By Ed Malaker
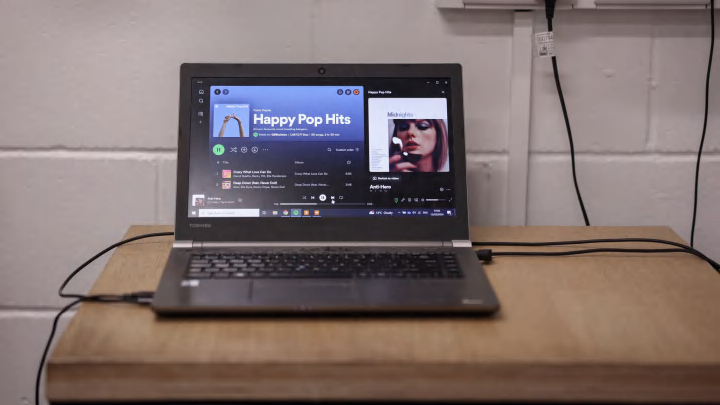
If you are looking for ways to increase the internet security of your devices, a simple port checker that you can build with Python is a great tool. Port checking, also called port scanning, is a method used to detect pen ports and services available on a networked computer to detect potential vulnerabilities. We’re going to show you how to build one and use it to protect your computer.
What is Port Checking?
Port checking involves sending packets to specific ports on a target machine to determine if they are open. Improperly managed ports on your computer can lead to spoofing, which is when another party impersonates your computer. SQL injection, cross-site request forgery, and denial of service attacks are just some of the tools a hacker can use against your system once they learn about open ports.
Use Python To Create A Port Checker
Using Python to create a port scanner is incredibly easy. We’ll give you the complete code at the end, but first, let’s see how it works. This guide assumes that you already have Python installed and have created some simple scripts. If you need help getting started, check out our guide to installing Python and starting your first projects. We also have a guide helping you choose an IDE that will make writing the code easier.
Import Required Libraries
The first thing we will need to do is import the required libraries into Python, and for this script, we will need to import “socket,” and “datetime.”
import socket
from datetime import datetime
Define the Target
Next, we will need the target. To scan your computer and look for open ports, type “ipconfig” into your command line and look for the “IPv4 Address” line in the response. Copy that into the code below.
target = input("Enter the target IP or hostname: ")
Initialize the Port Scanner
Next, we will tell the port checker which ports to scan. For this experiment, we will scan the first 1,024, which are the most common, but the number goes up to more than 65,000.
def port_scanner(target):
# Translate hostname to IPv4
target_ip = socket.gethostbyname(target)
print(f"Scanning target: {target_ip}")
print("Starting scan at:", datetime.now())
# Scan ports from 1 to 1024
for port in range(1, 1025):
scan_port(target_ip, port)
print("Scan completed at:", datetime.now())
Create the Port Scanning Function
The port scanning function is the part of our script that does the work, checking each port and signaling if it is open or there is an error.
def scan_port(ip, port):
try:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.setdefaulttimeout(1) # Set timeout to 1 second
result = sock.connect_ex((ip, port))
if result == 0:
print(f"Port {port}: Open")
sock.close()
except Exception as e:
print(f"Error scanning port {port}: {e}")
Run the Scanner
The final lines of code are what put it into motion.
if __name__ == "__main__":
port_scanner(target)
Full Script
Here is the full script that you can copy and paste.
import socket
from datetime import datetime
def scan_port(ip, port):
try:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.setdefaulttimeout(1)
result = sock.connect_ex((ip, port))
if result == 0:
print(f"Port {port}: Open")
sock.close()
except Exception as e:
print(f"Error scanning port {port}: {e}")
def port_scanner(target):
target_ip = socket.gethostbyname(target)
print(f"Scanning target: {target_ip}")
print("Starting scan at:", datetime.now())
for port in range(1, 1025):
scan_port(target_ip, port)
print("Scan completed at:", datetime.now())
if __name__ == "__main__":
target = input("Enter the target IP or hostname: ")
port_scanner(target)
Notes
Running your port checker can take some time because it has to send packets and wait for a response. It also only tells you about open ports, and if there are none, it’s easy to think it’s frozen. However, it will tell you when the scan is complete.
Using the Port Checker to Protect Your Computer
Run the port checker on your computer to learn about open ports and determine which ones are necessary with the help of the Service Name and Transport Protocol Port Number Registry. Close unnecessary ports using your firewall to prevent hackers from accessing your system. It’s also a good idea to scan the system regularly to ensure there are no changes.
If you enjoyed this guide and want to improve your security even more, check out our guide to using Python to encrypt your files.
feed