Harness the Power of APIs with Python: A Step-by-Step Guide for Beginners
By Ed Malaker
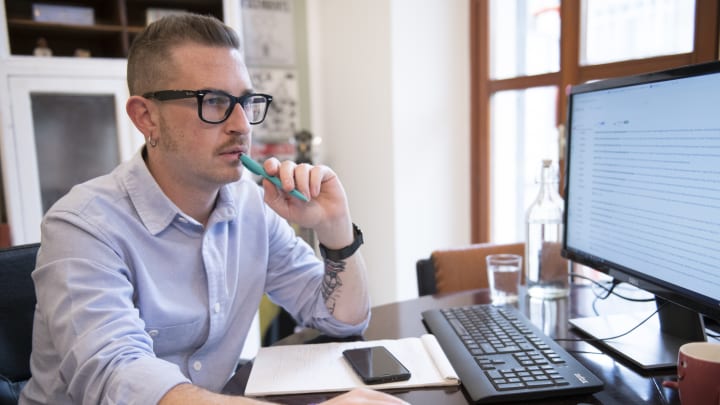
APIs (Application Programming Interfaces) are powerful tools that allow programs you create to interact with external services, which can significantly enhance their usability without requiring a lot of work. We see it being used a lot with ChatGPT, and a great way to learn how to start using an API is with Python.
What is an API?
An API, or Application Programming Interface, defines the methods and data formats that applications can use to request and exchange information with another application.
Components of an API
Endpoints
Endpoints are URLs provided by the API through which you can access its functionality, and each will have a different function.
Requests and Responses
A request is when you send a request to an endpoint, which can include parameters and data. The response is what you get back, and it will usually be in an XML or JSON format.
Methods
Methods are what you use for requests using an API and can include GET, POST, PUT, and DELETE.
Authentication
Most APIs require you to sign up to ensure that only authorized users can access the data. After you sign up, you will receive a key that you add to your programming code to access the data.
Rate Limiting
To prevent abuse, APIs often have rate limits, restricting the number of requests a user can make within a certain period, especially for free APIs, and exceeding this limit can result in additional fees.
How Can I Use an API?
To demonstrate how to use an API, we are going to create a small Python script to access the REST Countries API, which doesn’t require a key or a subscription, so that everyone can give it a try. We’ll break down and explain the code first, then provide the complete code you can copy and paste.
This guide assumes you already have Python installed and know how to use the scripts. Check out our Getting Started with Python guide if you are a beginner.
Setting Up the Environment
Before we can get started writing the script, you’ll need to install the requests library from the command prompt if you don’t already have it.
pip install requests
Fetching Data from the API
The first thing we will do is write a function to fetch the available data from the API. We’ll use a get request, and if successful, the API will return a JSON response.
import requests
# Function to fetch data from the REST Countries API
def fetch_country_data():
url = "https://restcountries.com/v3.1/all"
response = requests.get(url)
if response.status_code == 200:
return response.json()
else:
return None
Getting Country Information
Next, we’ll create a Python function to sort through the data to extract information about a country based on the user’s input.
# Function to get country information
def get_country_info(country_name, country_data):
for country in country_data:
if country_name.lower() in (country['name']['common'].lower(), country['name']['official'].lower()):
return {
'Name': country['name']['common'],
'Official Name': country['name']['official'],
'Population': country['population'],
'Capital': country.get('capital', ['N/A'])[0],
'Region': country['region'],
'Subregion': country.get('subregion', 'N/A'),
'Languages': ', '.join(country.get('languages', {}).values()),
'Currencies': ', '.join([currency['name'] for currency in country.get('currencies', {}).values()]),
'Flag URL': country['flags']['png']
}
return None
Displaying Country Information
Next, we need a function that displays the information we need on screen.
# Function to display country information
def display_country_info(country_info):
if country_info:
print("\nCountry Information:")
for key, value in country_info.items():
print(f"{key}: {value}")
else:
print("Country not found.")
Main Program Loop
Finally, we’ll need the main function that will continuously prompt the user for a country name and display the information until the user decides to exit.
# Main program
def main():
print("Welcome to the Country Information Program!")
country_data = fetch_country_data()
if not country_data:
print("Error fetching data from the API.")
return
while True:
country_name = input("\nEnter the name of the country (or type 'exit' to quit): ").strip()
if country_name.lower() == 'exit':
break
country_info = get_country_info(country_name, country_data)
display_country_info(country_info)
print("Thank you for using the Country Information Program!")
if __name__ == "__main__":
main()
Complete Code
import requests
# Function to fetch data from the REST Countries API
def fetch_country_data():
url = "https://restcountries.com/v3.1/all"
response = requests.get(url)
if response.status_code == 200:
return response.json()
else:
return None
# Function to get country information
def get_country_info(country_name, country_data):
for country in country_data:
if country_name.lower() in (country['name']['common'].lower(), country['name']['official'].lower()):
return {
'Name': country['name']['common'],
'Official Name': country['name']['official'],
'Population': country['population'],
'Capital': country.get('capital', ['N/A'])[0],
'Region': country['region'],
'Subregion': country.get('subregion', 'N/A'),
'Languages': ', '.join(country.get('languages', {}).values()),
'Currencies': ', '.join([currency['name'] for currency in country.get('currencies', {}).values()]),
'Flag URL': country['flags']['png']
}
return None
# Function to display country information
def display_country_info(country_info):
if country_info:
print("\nCountry Information:")
for key, value in country_info.items():
print(f"{key}: {value}")
else:
print("Country not found.")
# Main program
def main():
print("Welcome to the Country Information Program!")
country_data = fetch_country_data()
if not country_data:
print("Error fetching data from the API.")
return
while True:
country_name = input("\nEnter the name of the country (or type 'exit' to quit): ").strip()
if country_name.lower() == 'exit':
break
country_info = get_country_info(country_name, country_data)
display_country_info(country_info)
print("Thank you for using the Country Information Program!")
if __name__ == "__main__":
main()
What's Next?
This code only demonstrates a sample of what the REST Countries API offers. To help you understand the code better, try to modify it to use some of the other available data.
Follow GeekSided for more help using APIs and to leave comments and questions.
manual